Introduction
Flask is a micro web framework for Python, designed to be lightweight and modular while still offering the flexibility needed to build robust web applications. It is widely used for its simplicity, scalability, and extensive community support. This guide will take you from the very basics of Flask to advanced features, ensuring a solid understanding of the framework.
1. What is Flask?
Flask is a web framework for Python that provides tools, libraries, and technologies for building web applications. Unlike Django, which is a full-fledged web framework with built-in features, Flask follows a minimalistic approach, allowing developers to choose their tools as needed.
Features of Flask:
- Lightweight & Simple: Does not come with built-in ORM, authentication, or admin panel.
- Modular: Allows integration of extensions as per project needs.
- Flexible: Supports RESTful API development.
- Jinja2 Templating: Provides powerful templating for rendering dynamic HTML pages.
- WSGI-based: Uses Werkzeug, a WSGI toolkit for request handling.
2. Setting Up Flask
Installation
To get started, install Flask using pip:
pip install flask
Creating a Simple Flask Application
Create a Python file, e.g., app.py
, and write the following code:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return "Hello, Flask!"
if __name__ == '__main__':
app.run(debug=True)
Running the Flask App
python app.py
Navigate to http://127.0.0.1:5000/
in your browser to see the output.
3. Routing in Flask
Flask provides routing functionality to map URLs to functions.
@app.route('/about')
def about():
return "This is the about page."
Dynamic Routing
@app.route('/user/<string:name>')
def greet(name):
return f"Hello, {name}!"
URL Converters in Flask
Flask allows type-specific URL converters:
@app.route('/post/<int:post_id>')
def show_post(post_id):
return f"Post ID: {post_id}"
Using Multiple Routes
@app.route('/contact')
@app.route('/support')
def contact():
return "Contact us at support@example.com"
Handling 404 Errors
@app.errorhandler(404)
def page_not_found(e):
return "Page not found", 404
4. Flask Templates with Jinja2
Flask uses Jinja2 for rendering dynamic content in HTML.
Creating an HTML Template
Create a templates
directory and add index.html
inside:
<!DOCTYPE html>
<html>
<head>
<title>Home</title>
</head>
<body>
<h1>Welcome, {{ name }}!</h1>
</body>
</html>
Rendering the Template
from flask import render_template
@app.route('/welcome/<string:name>')
def welcome(name):
return render_template('index.html', name=name)
Using Control Structures in Jinja2
<ul>
{% for item in items %}
<li>{{ item }}</li>
{% endfor %}
</ul>
Extending Templates
Create base.html
:
<!DOCTYPE html>
<html>
<head>
<title>{% block title %}My Site{% endblock %}</title>
</head>
<body>
<nav>My Navigation Bar</nav>
{% block content %}{% endblock %}
</body>
</html>
Extend in another template:
{% extends "base.html" %}
{% block title %}Home{% endblock %}
{% block content %}
<h1>Welcome to my site!</h1>
{% endblock %}
5. Handling Forms and User Authentication
To handle user input, Flask provides the request
object.
from flask import request
@app.route('/login', methods=['GET', 'POST'])
def login():
if request.method == 'POST':
username = request.form['username']
return f"Welcome, {username}"
return '''<form method="post">Username: <input type="text" name="username"><input type="submit"></form>'''
User Authentication with Flask-Login
from flask_login import LoginManager, UserMixin, login_user, logout_user
login_manager = LoginManager()
login_manager.init_app(app)
class User(UserMixin):
pass
6. Flask with Databases (SQLAlchemy)
Creating and Connecting a Database
from flask_sqlalchemy import SQLAlchemy
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///data.db'
db = SQLAlchemy(app)
Creating Models
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(100))
Fetching Data from Database
@app.route('/users')
def get_users():
users = User.query.all()
return {"users": [user.name for user in users]}
7. Advanced Backend Concepts in Flask
Session Management
from flask import session
@app.route('/set_session')
def set_session():
session['username'] = 'JohnDoe'
return "Session set!"
JWT Authentication
from flask_jwt_extended import JWTManager, create_access_token
app.config['JWT_SECRET_KEY'] = 'secret'
jwt = JWTManager(app)
@app.route('/token')
def get_token():
return {"token": create_access_token(identity='user')}
Conclusion
Flask is a powerful framework that provides the flexibility to develop everything from simple web pages to complex APIs. This guide covered everything from setup to deployment, authentication, databases, error handling, middleware, caching, WebSockets, and background tasks, providing a strong foundation for working with Flask.
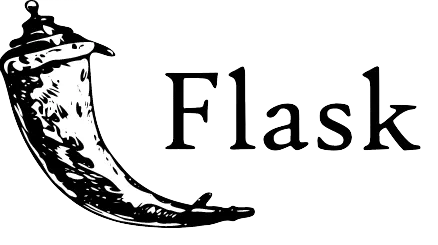
Leave a Reply